Bài giảng Ngôn ngữ lập trình Java - Bài 4: Lớp (Classes) và kế thừa (Inheritance)
- Lệnh return
public boolean isEmpty() { return items.isEmpty(); }
- Nếu method khai báo kiểu trả về là void, ta dùng lệnh return không có biểu thức hoặc là không cần lệnh return.
- Với override method, kiểu trả về có thể là subclass của kiểu trả về của overrided method (covariant return type Java 1.5) chứ không cần phải giống hoàn toàn.
Bạn đang xem 20 trang mẫu của tài liệu "Bài giảng Ngôn ngữ lập trình Java - Bài 4: Lớp (Classes) và kế thừa (Inheritance)", để tải tài liệu gốc về máy hãy click vào nút Download ở trên
Tóm tắt nội dung tài liệu: Bài giảng Ngôn ngữ lập trình Java - Bài 4: Lớp (Classes) và kế thừa (Inheritance)
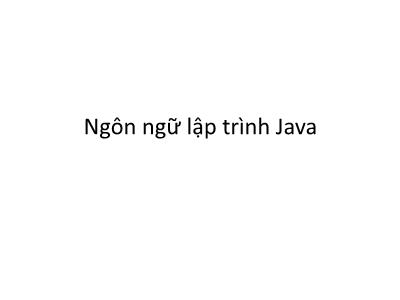
Ngôn ngữ lập trình Java Bài 4: Lớp (Classes) và kế thừa (Inheritance) Tạo lớp Khai báo lớp Element Function @annotation (Optional) An annotation (sometimes called meta-data) public (Optional) Class is publicly accessible abstract (Optional) Class cannot be instantiated final (Optional) Class cannot be subclassed class NameOfClass Name of the class (Optional) Comma-separated list of type variables extends Super (Optional) Superclass of the class implements Interfaces (Optional) Interfaces implemented by the class { ClassBody } Provides the class's functionality Khai báo Member Variables Element Function accessLevel (Optional) Access level for the variable static (Optional) Declares a class variable final (Optional) Indicates that the variable's value cannot change transient (Optional) Indicates that the variable is transient volatile (Optional) Indicates that the variable is volatile type name The type and name of the variable Định nghĩa Methods (1) Định nghĩa Methods (2) Element Function @ annotation (Optional) An annotation (sometimes called meta-data) accessLevel (Optional) Access level for the method static (Optional) Declares a class method (Optional) Comma-separated list of type variables. abstract (Optional) Indicates that the method must be implemented in concrete subclasses. final (Optional) Indicates that the method cannot be overridden native (Optional) Indicates that the method is implemented in another language synchronized (Optional) Guarantees exclusive access to this method returnType methodName The method's return type and name ( paramList ) The list of arguments to the method throws exceptions (Optional) The exceptions thrown by the method Định nghĩa Constructors Constructor có cùng tên với lớp. Không có kiểu trả về. Có hoặc không có tham số. public Stack() { } public Stack(int size) {} Trong constructor, ta có thể gọi superclass constructor: super([danh sách đối số]); hoặc constructor khác: this([danh sách đối]). Những lệnh này, nếu có, phải là lệnh đầu tiên trong constructor. Truyền tham số cho Methods, Constructors Java truyền tham số bằng giá trị: primitive type – passed by value; còn lại - passed by value of reference. Java 1.5 cho phép phương thức có thể nhận một số bất kỳ tham số (được gọi là varargs). public static Polygon polygonFrom(Point listOfPoints) { //listOfPoints kiểu Point[] } System.out.printf(String format, Object args); Trả về giá trị từ Methods Lệnh return . public boolean isEmpty() { return items.isEmpty(); } Nếu method khai báo kiểu trả về là void, ta dùng lệnh return không có biểu thức hoặc là không cần lệnh return . Với override method, kiểu trả về có thể là subclass của kiểu trả về của overrided method (covariant return type Java 1.5) chứ không cần phải giống hoàn toàn. Sử dụng từ khóa this Có ích khi ta cần truy cập đến các members của lớp mà trong phạm vi hiện thời có biến trùng tên: public class HSBColor { private int hue, saturation, brightness; public HSBColor (int hue, int saturation, int brightness) { this.hue = hue; this.saturation = saturation; this.brightness = brightness; } } Kiểm soát truy cập đến members của lớp Ta sử dụng access modifier. class Point { private int x, y; public int getX() { return x;} } Specifier Class Package Subclass World private Y N N N no specifier Y Y N N protected Y Y Y N public Y Y Y Y Members của instance và members của lớp Members của instance: không có từ khóa static. Chỉ truy cập được khi đối tượng được khới tạo. Members của lớp: khai báo có từ khóa static. Có thể truy cập trực tiếp qua tên lớp. class A { public void instanceMethod() {} public static void classMethod() {} } A a = new A(); a.instanceMethod(); a.classMethod(); A.classMethod(); Khởi tạo members của instance và members của lớp (1) Khởi tạo trực tiếp. private int i = 10; private static int count = 0; Khởi tạo instance member qua constructor hoặc initialization block – khối lệnh tự do trong body của class, được gọi trước mỗi constructor: { i = 10; } Khởi tạo members của instance và members của lớp (2) Khởi tạo class member qua static initialization block static { count = 0; } static initialization block được gọi thực hiện khi class được nạp vào hệ thống. Quản lý kế thừa Overriding và Hiding Methods Một instance method của subclass với cùng chữ ký (signature) và return type với instance method của superclass được gọi là overrides. Java 1.5 cho phép kiểu trả về của override method là kiểu con của kiểu trả về của method lớp cha (covariant return type). Instance method chỉ có thể override bằng một instance method. Static method chỉ có thể hide bằng một static method. Ngược lại, phát sinh lỗi compile. Hiding Member Variables Ta có thể định nghĩa một biến trong subclass trùng tên với biến trong superclass. Khi đó biến trong subclass sẽ che biến trong superclass. Để truy cập đến biến trong superclass phải dùng: super.variableName . Sử dụng từ khóa super Ta sử dụng từ khóa super để truy cập đến các phương thức, biến của lớp cha: super.memberName ; public class Superclass { public boolean aVariable; public void aMethod() { aVariable = true; } } public class Subclass extends Superclass { public boolean aVariable; //hides aVariable in Superclass public void aMethod() { //overrides aMethod in Superclass aVariable = false; super.aMethod(); System.out.format("%b%n", aVariable); System.out.format("%b%n", super.aVariable ); } } Final Class và Final Method Ta không thể extends một final class: public class final A {} public class B extends A {} //compile error Ta không thể override một final method: class A { final void finalMethod() {} } class B extends A { void finalMethod() {} //compile error } Abstract Method và Abstract Class Abstract method: chỉ có khai báo, không có body. abstract void draw(); Abstract class: khai báo với từ khóa abstract. public abstract class Figure {} Khi một lớp có 1 phương thức là abstract (hoặc chưa được implement), nó buộc phải khai báo là abstract. (nhưng ngược lại thì không đúng – một lớp abstract có thể không có một phương thức abstract nào). Những lớp con có nhiệm vụ implements các abstract methods. Ta không thể khởi tạo object của abstract class, chỉ có thể khai báo biến kiểu abstract class. Nested Classes Các loại nested class Static nested class Inner (non-static) class: new Parent().new Inner(); Local class Anonymous class Type Scope Inner static nested class member no inner [non-static] class member yes local class local yes anonymous class only the point where it is defined yes Kiểu liệt kê Sơ lược về kiểu liệt kê enum Là tập hợp cố định các constants. Dạng đơn giản nhất: enum Days { SUNDAY, MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY }; Lợi ích của kiểu liệt kê enum Có thể in ra tên có ý nghĩa. Typesafe Namespace Sử dụng trong lệnh switch Ta có thể định nghĩa thêm methods, fields; implements interface Implements Comparable, Serializable Phương thức quan trọng: values, name, ordinal, toString Tìm hiểu thêm: import static (Java 1.5), java.lang.Enum
File đính kèm:
bai_giang_ngon_ngu_lap_trinh_java_bai_4_lop_classes_va_ke_th.pptx