Bài giảng Lập trình Java cơ sở dữ liệu - Bài 3, Phần 1: Components - Nguyễn Hữu Thể
Nội dung
JLabel
JButton
JTextField
JCheckBox
JRadioButton
JPanel
JComboBox, JList
JTable
JMenu
JToolBar
JOptionPane
JFileChooser
Bạn đang xem 20 trang mẫu của tài liệu "Bài giảng Lập trình Java cơ sở dữ liệu - Bài 3, Phần 1: Components - Nguyễn Hữu Thể", để tải tài liệu gốc về máy hãy click vào nút Download ở trên
Tóm tắt nội dung tài liệu: Bài giảng Lập trình Java cơ sở dữ liệu - Bài 3, Phần 1: Components - Nguyễn Hữu Thể
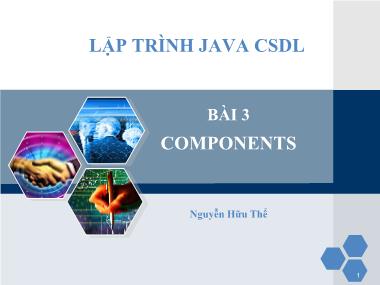
1 LẬP TRÌNH JAVA CSDL BÀI 3 COMPONENTS Nguyễn Hữu Thể 2 Nội dung JLabel JButton JTextField JCheckBox JRadioButton JPanel JComboBox, JList JTable JMenu JToolBar JOptionPane JFileChooser GUI Components JButton, JLabel, JTextField, JCheckBox, JRadioButton, and JComboBox. Each GUI component class provides several constructors that you can use to create GUI component objects. 3 GUI Components // Create a button with text OK JButton btOK = new JButton("OK"); // Create a label with text "Enter your name: " JLabel lbName = new JLabel("Enter your name: "); // Create a text field with text "Type Name Here" JTextField txtName = new JTextField("Type Name Here"); // Create a check box with text bold JCheckBox chkBold = new JCheckBox("Bold"); // Create a radio button with text red JRadioButton rbRed = new JRadioButton("Red"); // Create a combo box with choices red, green, blue JComboBox cboColor = new JComboBox(new String[]{"Red", "Green", "Blue"}); 4 Working with Components javax.swing.Jcomponent Several methods: setEnabled(boolean): receive user input (an argument of true) or is inactive and cannot receive input (false). Components are enabled by default. isEnabled() returns a boolean value. setVisible(boolean): for all components. Use true to display a component and false to hide it. isVisible() setSize(int, int): width and height specified as arguments setSize(Dimension) uses a Dimension getSize(): returns a Dimension object with height and width 5 Working with Components setText() getText() setValue() and getValue() for components that store a numeric value. 6 JLabel Display Text, not editable Constructor: Label() : An empty label JLabel(String) : A label with the specified text JLabel(String, int) : A label with the specified text and alignment LEFT, CENTER, and RIGHT. JLabel(String, Icon, int) : A label with the specified text, icon, and Alignment 7 JLabel Common Methods: void setFont (Font f) void setText(String S) String getText() void setIcon(Icon) 8 JLabel Example: JLabel lbl=new JLabel("Họ và tên:"); JLabel lbl=new JLabel("Ngày sinh:"); 9 JLabel The use of HTML is supported by most Swing components. Example: use HTML markup to create multiline and multifont labels: JLabel lbHoten = new JLabel("Dòng 1<p style=\"color:red;font-size:20\">Dòng 2"); 10 JTextField Display data, Input data Constructors JTextField(): An empty text field JTextField(int): A text field with the specified width JTextField(String): A text field with text JTextField(String, int): A text field with the specified text and width Common Methods void setText(String S) String getText() void setEditable(boolean b) boolean isEditable() JTextField Example setLayout(new FlowLayout()); JLabel lbHoten = new JLabel("Nhập họ và tên"); add(lbHoten); JTextField txtHoten = new JTextField(20); add(txtHoten); JTextField Don’t allow input data txtHoten.setEditable(false) To set Text in code behind for JTextField txtHoten.setText("Hello world"); To get Text from JTextField String s = txtHoten.getText(); We could convert data int n = Integer.parseInt(s); //s is String double d = Double.parseDouble(s); float f = Float.parseFloat(s); To set focus: txtHoten.requestFocus(); JPasswordField Hide the characters a user is typing into the field. JPasswordField class, a subclass of JTextField. JPasswordField constructor methods take the same arguments as those of its parent class. Methods: JPasswordField(String text, int columns) char[] getPassword(): returns the text contained in this password field JPasswordField setEchoChar(char): replacing each input character with the specified character JPasswordField pass = new JPasswordField(20); pass.setEchoChar('#'); JTextArea Input is more than one line long Constructors JTextArea(): Create a default text area. JTextArea(int rows, int columns): Create a text area with the specified number of rows and columns. JTextArea(String text) JTextArea(String text, int rows, int columns) JTextArea(Document doc): Create a text area that uses the specified Document. JTextArea(Document doc, String text, int rows, int columns) JTextArea Common methods void append(String str) Append the given text to the end of the document. void insert(String str, int pos) Insert the specified text at the given position . To insert text at the beginning of the document, use a position of 0. void replaceRange(String str, int start, int end) Replace a section of the document JTextArea public int getLineStartOffset(int line) throws BadLocationException Return the character offset (from the beginning) that marks the beginning of the specified line number. public int getLineEndOffset(int line) throws BadLocationException Return the character offset (from the beginning) that marks the end of the specified line number. This is actually the offset of the first character of the next line. public int getLineOfOffset(int offset) throws BadLocationException Return the line number that contains the given character offset (from the beginning of the document). JTextArea JPanel contentPane = new JPanel(); JLabel lblNewLabel = new JLabel("Nhập dữ liệu:"); contentPane.add(lblNewLabel); JTextArea textArea = new JTextArea(3,15); textArea.setWrapStyleWord(true); textArea.setLineWrap(true); JScrollPane scrollPane = new JScrollPane(textArea); contentPane.add(scrollPane); JTextArea class MyJTextArea extends JFrame { private JPanel contentPane; public MyJTextArea() { setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setBounds(100, 100, 257, 128); contentPane = new JPanel(); contentPane.setBorder(new EmptyBorder(5, 5, 5, 5)); setContentPane(contentPane); contentPane.setLayout(new FlowLayout(FlowLayout.CENTER, 5, 5)); JLabel lblNewLabel = new JLabel("Nhập dữ liệu:"); contentPane.add(lblNewLabel); JScrollPane scrollPane = new JScrollPane(); JTextArea textArea = new JTextArea(3,15); textArea.setWrapStyleWord(true); textArea.setLineWrap(true); scrollPane.setViewportView(textArea); contentPane.add(scrollPane); } } JButton JButton() Creates a button with no set text or icon. JButton(Action a) Creates a button where properties are taken from the Action supplied. JButton(Icon icon) Creates a button with an icon. JButton(String text) Creates a button with text. JButton(String text, Icon icon) Creates a button with initial text and an icon. JButton AccessibleContext getAccessibleContext() Gets the AccessibleContext associated with this JButton. String getUIClassID() Returns a string that specifies the name of the L&F class that renders this component. boolean isDefaultButton() Gets the value of the defaultButton property, which if true means that this button is the current default button for its JRootPane. boolean isDefaultCapable() Gets the value of the defaultCapable property. protected String paramString() Returns a string representation of this JButton. JButton void removeNotify() Overrides JComponent.removeNotify to check if this button is currently set as the default button on the RootPane, and if so, sets the RootPane's default button to null to ensure the RootPane doesn't hold onto an invalid button reference. void setDefaultCapable(boolean defaultCapable) Sets the defaultCapable property, which determines whether this button can be made the default button for its root pane. void updateUI() Resets the UI property to a value from the current look and feel. JButton 24 It is very important, attach event to do something that you want. JButton bt=new JButton("Watch"); bt.setIcon(new ImageIcon("mywatch.png")); bt.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent arg0) { //do something here coding here } }); Add event for this button bt.setMnemonic('W'); Alt+W to call bt command JButton JRadioButton JRadioButton() Creates an initially unselected radio button with no set text. JRadioButton(Action a) Creates a radiobutton where properties are taken from the Action supplied. JRadioButton(Icon icon) Creates an initially unselected radio button with the specified image but no text. JRadioButton(Icon icon, boolean selected) Creates a radio button with the specified image and selection state, but no text. JRadioButton(String text) Creates an unselected radio button with the specified text. JRadioButton(String text, boolean selected) JRadioButton(String text, Icon icon) Creates a radio button that has the specified text and image, and that is initially unselected. JRadioButton(String text, Icon icon, boolean selected) JRadioButton - Methods Modifier and Type Method and Description AccessibleContext getAccessibleContext()Gets the AccessibleContext associated with this JRadioButton. String getUIClassID()Returns the name of the L&F class that renders this component. protected String paramString()Returns a string representation of this JRadioButton. void updateUI()Resets the UI property to a value from the current look and feel. JRadioButton 28 Make single choice Must add JRadioButton into the ButtonGroup if(radio.isSelected()) { } JRadioButton 29 ButtonGroup & JRadioButton JPanel panelGroup=new JPanel(); panelGroup.setLayout(new BoxLayout(panelGroup, BoxLayout.Y_AXIS)); TitledBorder titlebor=new TitledBorder("Ý kiến của bạn:"); panelGroup.setBorder(titlebor); JRadioButton rad1=new JRadioButton("Lập trình Java rất dễ"); JRadioButton rad2=new JRadioButton("Design GUI Java rất dễ"); JRadioButton rad3=new JRadioButton("Viết web trên Java rất dễ"); JRadioButton rad4=new JRadioButton("Tất cả đều khó"); ButtonGroup group=new ButtonGroup(); group.add(rad1);group.add(rad2); group.add(rad3);group.add(rad4); panelGroup.add(rad1);panelGroup.add(rad2); panelGroup.add(rad3);panelGroup.add(rad4); add(panelGroup); JRadioButton & ButtonGroup 30 Create Border with title: Border bor=BorderFactory.createLineBorder(Color.RED); TitledBorder titlebor=new TitledBorder(bor,"Ý kiến của bạn:"); panelGroup.setBorder(titlebor); Define a buttongroup to add all radio: ButtonGroup group=new ButtonGroup(); group.add(rad1); And add all Radio into the pnGroup: panelGroup.add(rad1); Add panelGroup into the Window: add(panelGroup); Checkbox JCheckBox() Creates an initially unselected check box button with no text, no icon. JCheckBox(Action a) Creates a check box where properties are taken from the Action supplied. JCheckBox(Icon icon) Creates an initially unselected check box with an icon. JCheckBox(Icon icon, boolean selected) Creates a check box with an icon and specifies whether or not it is initially selected. JCheckBox(String text) Creates an initially unselected check box with text. JCheckBox(String text, boolean selected) JCheckBox(String text, Icon icon) JCheckBox(String text, Icon icon, boolean selected) Checkbox Make multi choice Set grid layout 2 rows and 2 column pnCheck.setLayout(new GridLayout(2, 2)); Create JCheckBox: JCheckBox chk1=new JCheckBox("Java"); Add chk1 into the pnCheck: pnCheck.add(chk1); Add pnCheck into the Window: add(pnCheck); BorderFactory.createEtchedBorder(Color.BLUE, Color.RED); Create border with 2 color: Blue, Red: if(chk1.isSelected()) { //do something } Checkbox setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setBounds(100, 100, 200, 150); panelCheck=new JPanel(); panelCheck.setLayout(new GridLayout(2, 2)); Border bor2=BorderFactory.createEtchedBorder(Color.BLUE, Color.RED); TitledBorder titlebor2=new TitledBorder(bor2, "Môn học yêu thích:"); panelCheck.setBorder(titlebor2); JCheckBox chk1=new JCheckBox("C/C++"); JCheckBox chk2=new JCheckBox("C#"); JCheckBox chk3=new JCheckBox("PHP"); JCheckBox chk4=new JCheckBox("Java"); panelCheck.add(chk1);panelCheck.add(chk2); panelCheck.add(chk3);panelCheck.add(chk4); add(panelCheck); JComboBox JComboBox() Creates a JComboBox with a default data model. JComboBox(ComboBoxModel aModel) Creates a JComboBox that takes its items from an existing ComboBoxModel. JComboBox(E[] items) Creates a JComboBox that contains the elements in the specified array. JComboBox(Vector items) Creates a JComboBox that contains the elements in the specified Vector. JComboBox JComboBox cbo=new JComboBox(); cbo.addItem("Xuất sắc"); cbo.addItem("Giỏi"); cbo.addItem("Khá"); cbo.addItem("Trung bình"); add(cbo); String arr[]={"Xuất sắc" ,"Giỏi" ,"Khá","Trung bình"}; JComboBox cbo=new JComboBox(arr); add(cbo); JComboBox int n=cbo.getSelectedIndex(); n is position that we selected Object o=cbo.getSelectedItem(); We could cast object to valid another type cbo.setSelectedIndex(-1); To clear selection
File đính kèm:
bai_giang_lap_trinh_java_co_so_du_lieu_bai_3_phan_1_componen.pdf